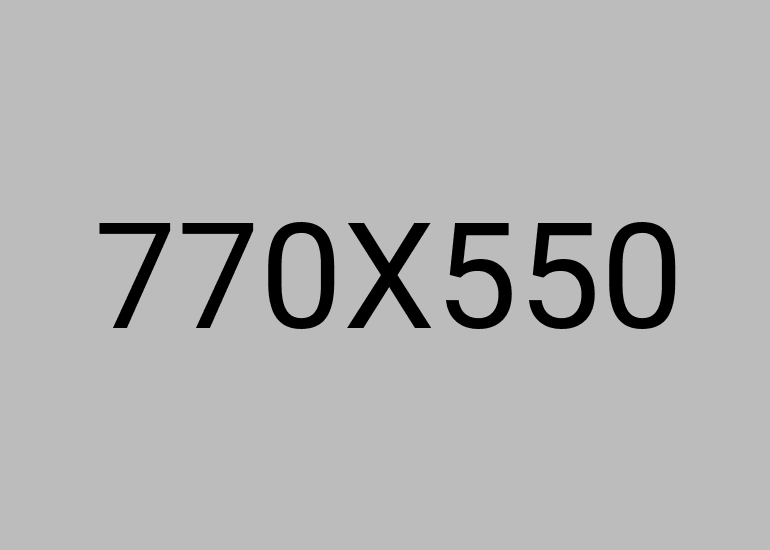
27 May 2023
Dependency injection is a design pattern that helps manage dependencies between different components of an application. It allows for better code organization, testability, and flexibility by reducing coupling between classes.
In Laravel, dependency injection is primarily handled through the service container, which is an integral part of the framework. The service container is responsible for resolving and managing dependencies across your application.
To use dependency injection in Laravel, you typically follow these steps:
Let's define the dependencies. You need to define the dependencies that your class or method requires. You can do this with constructor injection.
Сonstructor injection.
You can specify dependencies as constructor parameters.
Inside the EventController, define a constructor that accepts as an input parameter a EventService object.
The storeNewEvent() action method uses the $eventService object to perform the store newEvent.
When you send a request to the storeNewEvent() method, Laravel does many tasks behind the scenes. One of those tasks is to instantiate the EventController class. While instantiating it, it observes that the constructor requires a dependency.
The EventService class defines a constructor that accepts a dependency named $createEventHandler.
And then the CreateEventHandler class now defines a constructor that accepts a dependency named EventRepositoryInterface $eventRepository.
In this case, Laravel won't be able to instantiate the EventService on its own without some help from your side. The reason? Laravel cannot predict or provide the new dependency.
You must provide Laravel with additional instructions on how to instantiate the EventService.
Binding Dependencies: Laravel allows you to bind interfaces or abstract classes to their concrete implementations in the service container. This enables you to swap implementations easily or define custom resolution logic.
You can use a service provider to bind dependencies within the register method. Service providers are an excellent way to organize your bindings and other container-related configurations.
Inside the app\Providers\AppServiceProvider.php file, you register a binding to tell Laravel how to instantiate a new object of the EventService.
These are the basic steps involved in using dependency injection in Laravel. By leveraging Laravel's service container, you can manage your dependencies effectively and promote reusable, testable code throughout your application.